服务发现是微服务架构体系中最关键的组件之一。Nacos 除了可以作为配置中心,也可以用于服务发现,服务端将接口注册到 Nacos 中,消费端动态获取服务列表,使用特定的负载算法选择其中一个服务来完成服务的调用。在这篇文章里,使用官方的代码实例来学习 Nacos 服务发现功能。
实例介绍
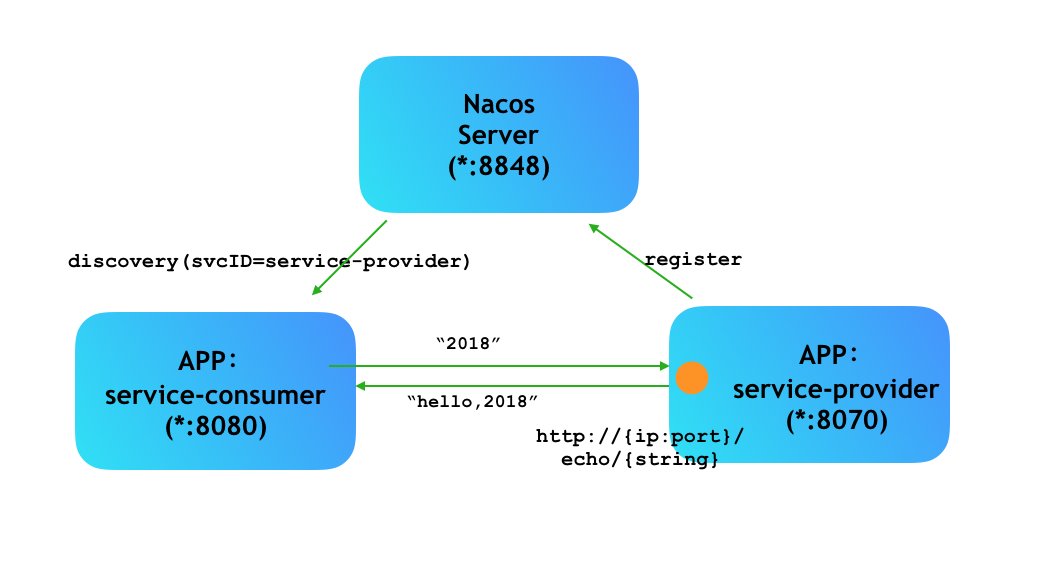
实例包含两个程序,一个是服务提供者用于实现特定的功能,是服务的提供者,一个是服务的消费者,是服务的调用方。
版本依赖
在这里我们使用 Spring Cloud Alibaba 来引入 Nacos, 它会依赖 Springboot 和 SpringCloud 的版本。三者的版本关系如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
| <properties> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target>
<spring-boot.version>2.2.5.RELEASE</spring-boot.version> <spring-cloud.version>Hoxton.SR3</spring-cloud.version> <spring-cloud-alibaba.version>2.2.1.RELEASE</spring-cloud-alibaba.version> </properties>
<dependencyManagement>
<dependencies>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-dependencies</artifactId> <version>${spring-boot.version}</version> <type>pom</type> <scope>import</scope> </dependency>
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency>
<dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-alibaba-dependencies</artifactId> <version>${spring-cloud-alibaba.version}</version> <type>pom</type> <scope>import</scope> </dependency>
</dependencies>
</dependencyManagement>
|
三者之间的版本依赖关系,可以在官网查看:版本说明 Wiki
引入 Nacos Discovery Starter
使用 Nacos Discovery Starter 引入 Nacos 的服务发现功能。
1 2 3 4
| <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId> </dependency>
|
配置服务提供者
配置服务提供者,可以将其服务注册到 Nacos server 上。
配置 application.yml(propertities)
设置服务器的端口、Nacos 的地址、用户名及密码信息。
1 2 3 4 5 6 7 8 9 10 11 12
| server: port: 8070
spring: application: name: service-provider cloud: nacos: discovery: server-addr: 127.0.0.1:8848 username: nacos password: nacos
|
开启服务发现
通过 Spring Cloud 原生注解 @EnableDiscoveryClient 开启服务注册发现功能
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| @SpringBootApplication @EnableDiscoveryClient public class NacosProviderApplication {
public static void main(String[] args) { SpringApplication.run(NacosProviderApplication.class, args); }
@RestController @RequestMapping("/services") class EchoController { @RequestMapping(value = "/echo/{string}", method = RequestMethod.GET) public String echo(@PathVariable String string) { return "Hello Nacos Discovery " + string; } } }
|
配置服务消费者
配置服务消费者,服务消费者可以通过 Nacos 的服务注册发现功能从 Nacos server 上获取到它要调用的服务。
配置 application.yml(propertities)
配置服务器的端口、Nacos 的地址、用户名及密码信息。
1 2 3 4 5 6 7 8 9 10 11 12
| server: port: 8080
spring: application: name: service-consumer cloud: nacos: discovery: server-addr: 127.0.0.1:8848 username: nacos password: nacos
|
开启服务发现
通过 Spring Cloud 原生注解 @EnableDiscoveryClient 开启服务注册发现功能。给 RestTemplate 实例添加 @LoadBalanced 注解,开启 @LoadBalanced 与 Ribbon 的集成:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| @SpringBootApplication @EnableDiscoveryClient public class NacosConsumerApplication {
@LoadBalanced @Bean public RestTemplate restTemplate() { return new RestTemplate(); }
public static void main(String[] args) { SpringApplication.run(NacosConsumerApplication.class, args); }
@RestController public class TestController {
private final RestTemplate restTemplate;
@Autowired public TestController(RestTemplate restTemplate) { this.restTemplate = restTemplate; }
@RequestMapping(value = "/echo/{str}", method = RequestMethod.GET) public String echo(@PathVariable String str) { return restTemplate.getForObject("http://service-provider/services/echo/" + str, String.class); } } }
|
启动并测试
启动 ProviderApplication 和 ConsumerApplication ,调用 http://localhost:8080/echo/2022,返回内容为 Hello Nacos Discovery 2022。
代码库:https://github.com/noahsarkzhang-ts/springboot-lab
参考:
1. Nacos Spring Cloud 快速开始
2. Spring Cloud Alibaba Nacos Discovery
2. Spring Cloud Alibaba 版本说明